Appearance
Introduction
Forge Actions are simply asynchronous functions that can:
- Collect input to be processed by your service
- Render output to display data on your forms
- Notify the user on change and submit events
- Trigger other actions
Defining Actions
The recommended pattern to define actions is via path-based routing. This allows you to group pages and actions as your Forge apps grow in complexity.
INFO
The Forge Python SDK currently does not support path-based routing. We plan to release this feature to our Python SDK in a future release.
ts
import { Forge } from '@forgeapp/sdk'
const forge = new Forge({
apiKey: '<YOUR_API_KEY>',
endpoint: 'wss://<FORGE_SERVER_URL>/websocket',
routesDirectory: path.resolve(__dirname, "routes"),
})
forge.listen()
Alternatively, you can define actions inline.
ts
import { Forge } from '@forgeapp/sdk'
import { refundOrder } from '../api/order'
const forge = new Forge({
apiKey: '<YOUR_API_KEY>',
endpoint: 'wss://<FORGE_SERVER_URL>/websocket',
actions: {
refundCustomerOrder: async () => {
const orderID = await io.input.text('Order ID')
await refundOrder(orderID)
return 'Success!'
},
},
})
forge.listen()
python
from forgeapp_sdk import Forge
from api import refund_order
forge = Forge(
api_key="<YOUR_API_KEY>",
endpoint="wss://<FORGE_SERVER_URL>/websocket",
)
@forge.action(name="Refund Customer Order")
async def refund_customer_order(io: IO):
order_id = await io.input.text("Order ID")
await refund_order(order_id)
return 'Success!'
forge.listen()
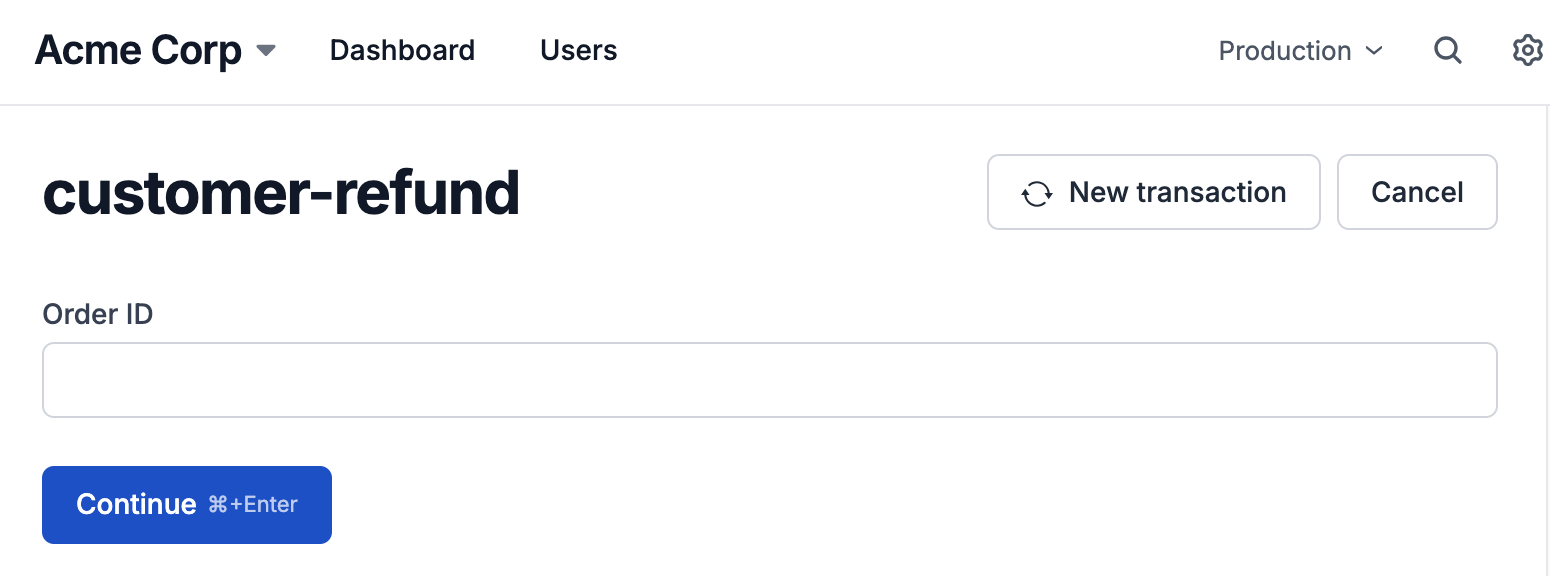