Appearance
Getting started
The Forge SDK provides developers with all the components they need to create Forge apps. To start building on the Forge platform, install our SDK
bash
npm i @forgeapp/sdk
bash
pip install forgeapp-sdk
Then, create your first Forge app in just a few lines of code.
ts
import { Forge } from '@forgeapp/sdk'
import { refundOrder } from '../api/order'
const forge = new Forge({
apiKey: '<YOUR_API_KEY>',
endpoint: 'wss://<FORGE_SERVER_URL>/websocket',
actions: {
refundCustomerOrder: async () => {
const orderID = await io.input.text('Order ID')
await refundOrder(orderID)
return 'Success!'
},
},
})
forge.listen()
python
from forgeapp_sdk import Forge
from api import refund_order
forge = Forge(
api_key="<YOUR_API_KEY>",
endpoint="wss://<FORGE_SERVER_URL>/websocket",
)
@forge.action(name="Refund Customer Order")
async def refund_customer_order(io: IO):
order_id = await io.input.text("Order ID")
await refund_order(order_id)
return 'Success!'
forge.listen()
🎉 That's it! Run your service and you'll be able to view your new Forge app in your Forge dashboard. When the user submits the action, the Forge server will call your function with the input data.
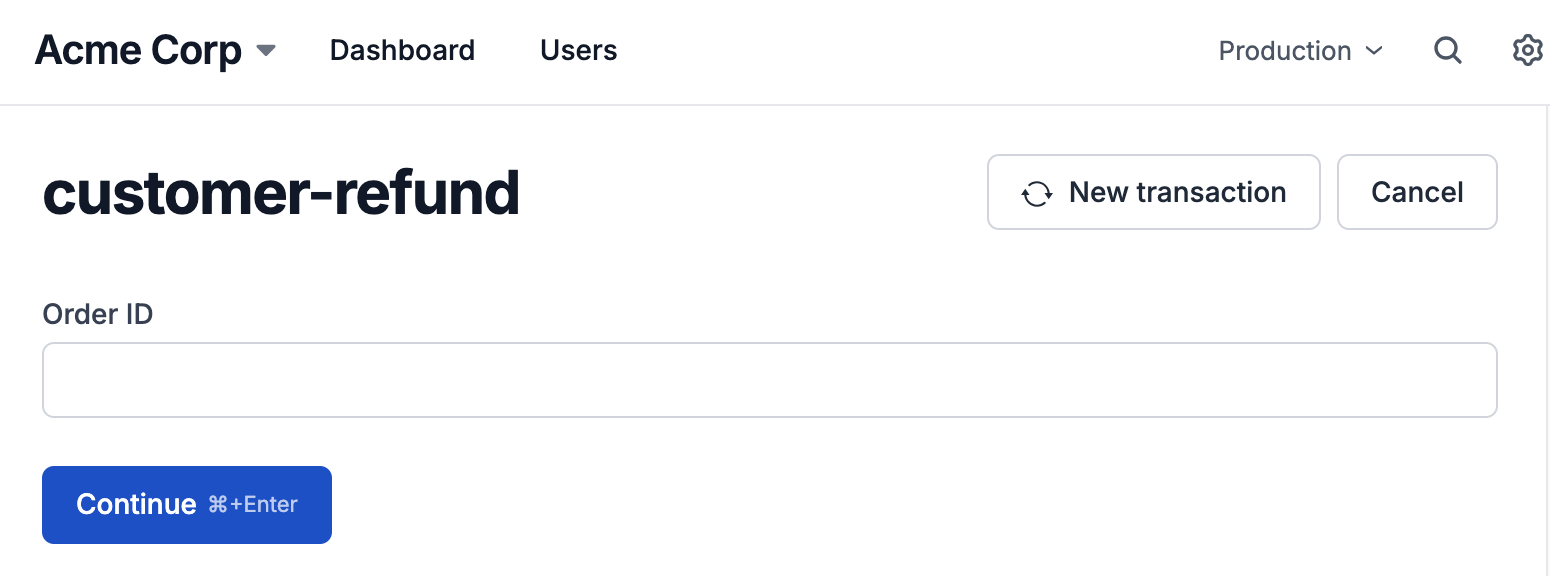