Appearance
io.select.single
Represents a select.single component.
Usage
ts
const { value } = await io.select.single("T-Shirt Size", {
options: [
{
label: "Small",
value: "sm",
},
{
label: "Medium",
value: "md",
},
{
label: "Large",
value: "lg",
},
{
label: "XLarge",
value: "xl",
},
],
defaultValue: "Size",
helpText: "Currently only S-XL is in stock",
})
python
value = await io.select.single(
"T-Shirt Size",
options=[
{
"label": "Small",
"value": "sm",
},
{
"label": "Medium",
"value": "md",
},
{
"label": "Large",
"value": "lg",
},
{
"label": "XLarge",
"value": "xl",
},
],
default_value="Size",
help_text="Currently only S-XL is in stock"
)
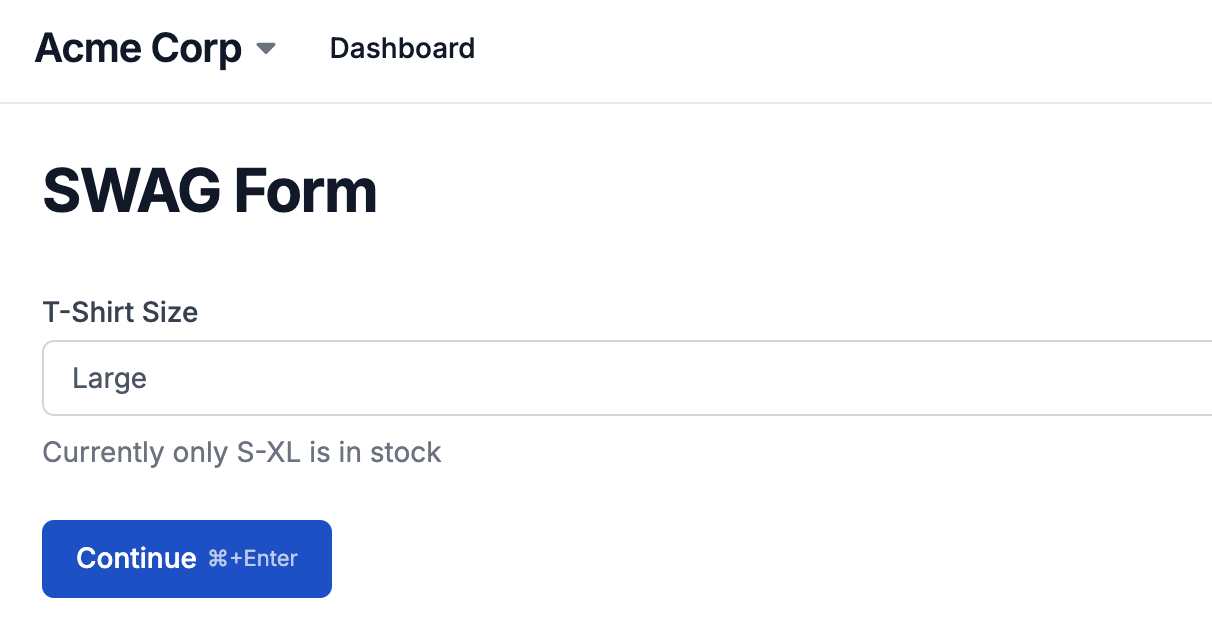
Props
defaultValue Optional
string | number | boolean | Date | object
Default preselected option. Must be one of the items in options
.
disabled Optional
boolean
Whether the input is disabled, preventing changes from the defaultValue
.
helpText Optional
string
Secondary label providing additional context for the selection. Supports inline markdown elements like bold, italics, and links.
options Required
(string | number | boolean | Date | object)[]
Array of possible values to be selected. Can be an array of primitive values, or provide objects for more advanced customization.
description Optional
string
Secondary label to provide additional context for the option.
image Optional
object
Image to render alongside the option.
{
// a URL to the image
url?: string
// the image alt tag
alt?: string
// the size of the image
size?: "thumbnail" | "small" | "medium" | "large"
}
imageUrl Optional
string
Deprecated, replaced with image.url
.
label Required
string | number | boolean | Date
Display label for this particular possible option.
value Required
string | number | boolean | Date
Value for this particular possible option.
searchable Optional
boolean
Whether the select should support filtering options via a search box.
Returns
The selected option.
Examples
String options
The options
property can also be an array of strings, which is equivalent to objects with identical label and value properties with the string values. A string will be returned if this form is used.
const currencyCode = await io.select.single("Currency", {
options: ["USD", "CAD", "EUR"],
defaultValue: "USD",
helpText: "Currency for this transaction",
});